상속
상속 - 기존의 클래스를 재사용해서 새로운 클래스를 작성하는 것
class 자손클래스 extends 조상클래스 {}
- 자손은 조상의 모든 멤버를 상속받음(생성자, 초기화블럭 제외)
포함 - 한 클래스의 멤버변수로 다른 클래스를 선언하는 것.
class Circle {
Point c = new Point();
int r;
관계설정 방법
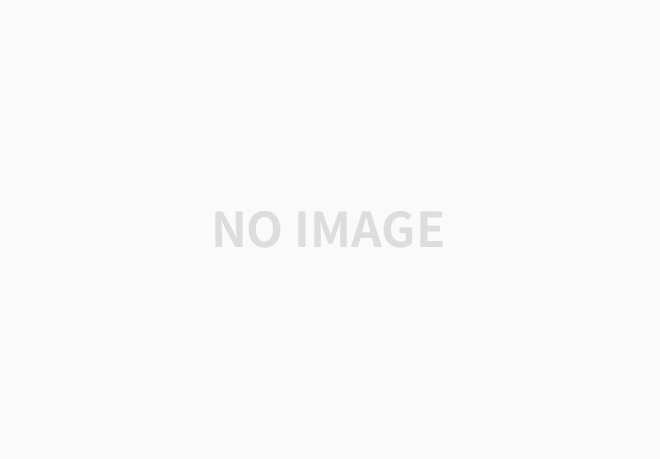
단일상속 - java는 단일상속만을 하용한다.
- 비중이 높은 클래스는 상속관계, 나머지는 포함관계
class TVCR extends TV,VCR //불가
Object 클래스 - 모든 클래스의 최고조상
- 모든 클래스는 Object클래스를 상속받고 조상이 없는 클래스는 자동적으로 Object 클래스를 상속받는다.
오버라이딩
오버라이딩 - 조상클래스로부터 상속받은 메서드의 내용을 상속받는 클래스에 맞게 변경하는것.
Point3D p = new Point3D();
p.x = 1;
p.y = 2;
System.out.println(p.getLocation());
}
}
class Point {
int x;
int y;
String getLocation() {
return x + "," + y;
}
}
class Point3D extends Point {
int y;
String getLocation() {
return "extends" + x + "," + y;
}
}
조건
- 선언부가 같아야함 ( 이름,매개변수,리턴타입)
- 접근제어자를 좁은범위로 변경 불가 ( protected면 넓은 protected 나 public가능)
- 조상클래스의 메서드보다 많은 수의 예외를 선언할 수 없다.
오버로딩 vs 오버라이딩
class Parent {
void parentMethod() {}
}
class child extends Parent {
void parentMethod() {} // 오버라이딩
void parentMethod(int i) {} //오버로딩
}
super
this - 인스턴스 자신을 가리키는 참조변수 , 지역변수와 인스턴스변수를 구분하기 위해
super - this 와 같음. 조상의 멤버와 자신의 멤버를 구별하는데 사용.
class Parent{
int x=10;
}
class child extends Parent {
int x=20;
void methid() {
System.out.println(x); //지역변수
System.out.println(this.x); /인스턴스 변수
System.out.println(super.x); // 조상의 변수
}
}
조상 클래스의 메서드 호출
class Point {
int x;
int y;
String getLocation() {
return x + "," + y;
}
}
class Point3D extends Point {
int y;
String getLocation() {
//return "extends" + x + "," + y;
return super.getLocation() + ",";
}
}
super()
조상의 생성자
- 자손클래스의 인스턴스를 생성하면 자손의 멤버와 조상의 멤버가 합쳐진 하나의 인스턴스가 생성
- 자손의 생성자 첫문장에서 조상의 생성자를 호출해야한다. ( 없으면 자동으로 super();를 삽입함.)
class Point {
int x;
int y;
Point() {}
Point(int x, int y) {
this.x = x;
this.y = y;
}
String getLocation() {
return x + "," + y;
}
}
class Point3D extends Point {
int z;
Point3D(int x, int y , int z) {
super(x,y);
this.z = z;
}
패키지
- 서로 관련된 클래스와 인터페이스의 묶음
- 클래스의 실제이름은 패키지명이 포함된것 ( Stirng클래스의 이름은 java.lang.String)
- 패키지는 소스파일에 첫번째 문장에 선언
package 패키지명
import 패키지명
'IT 관련 > JAVA' 카테고리의 다른 글
자바 객체지향II (추상화, 인터페이스) (0) | 2022.06.30 |
---|---|
자바 객체지향언어II(제어자 ,다형성) (0) | 2022.06.29 |
자바 객체지향언어I (생성자 , 변수의 초기화) (0) | 2022.06.23 |
자바 객체지향언어I (재귀, 클래스메서드와 인스턴스메서드, 메서드 오버로딩) (0) | 2022.06.22 |
자바 객체지향언어I (JVM메모리 , 기본형 참조형 매개변수) (0) | 2022.06.17 |